本文源码请见我的GitHub
1 | import pandas as pd |
1 | rainfall = pd.read_csv('./Seattle2014.csv') |
1 | rainfall =rainfall['PRCP'].values |
1 | inches = rainfall / 254 #1/1mm ->inches |
(365,)
1 | %matplotlib inline |
1 | plt.hist(inches, 40); |
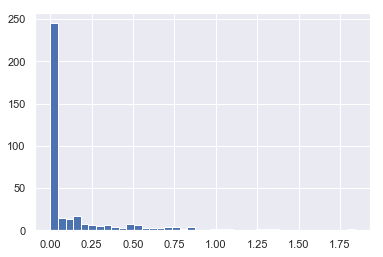
2.6.2 和通用函数类似的比较操作
1 | x = np.array([1, 2, 3, 4, 5]) |
1 | x < 3 |
array([ True, True, False, False, False])
1 | x > 3 |
array([False, False, False, True, True])
1 | x <= 3 |
array([ True, True, True, False, False])
1 | x!=3 |
array([ True, True, False, True, True])
1 | x == 3 |
array([False, False, True, False, False])
1 | (2 * x) == (x ** 2) |
array([False, True, False, False, False])
1 | rng = np.random.RandomState(0) |
array([[5, 0, 3, 3],
[7, 9, 3, 5],
[2, 4, 7, 6]])
1 | x < 6 |
array([[ True, True, True, True],
[False, False, True, True],
[ True, True, False, False]])
2.6.3 布尔数组
1 | print(x) |
[[5 0 3 3]
[7 9 3 5]
[2 4 7 6]]
1 | np.count_nonzero(x < 6) |
8
1 | np.sum(x < 6) |
8
1 | np.sum(x < 6, axis=1) |
array([4, 2, 2])
1 | np.any(x > 8) |
True
1 | np.any(x < 0) |
False
1 | np.all(x < 10) |
True
1 | np.all(x == 6) |
False
1 | np.all(x < 8, axis = 1) |
array([ True, False, True])
1 | np.sum((inches > 0.5) | (inches >= 1)) |
37
1 | print("number days without rain: ",np.sum(inches == 0)) |
number days without rain: 215
number days with rain 150
days with more than 0.5 inches: 37
2.6.4 江布尔数组作为掩码
1 | x |
array([[5, 0, 3, 3],
[7, 9, 3, 5],
[2, 4, 7, 6]])
1 | x < 5 |
array([[False, True, True, True],
[False, False, True, False],
[ True, True, False, False]])
1 | x[x<5] |
array([0, 3, 3, 3, 2, 4])